Crud operations using Mongodb and Mongoose
Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js.
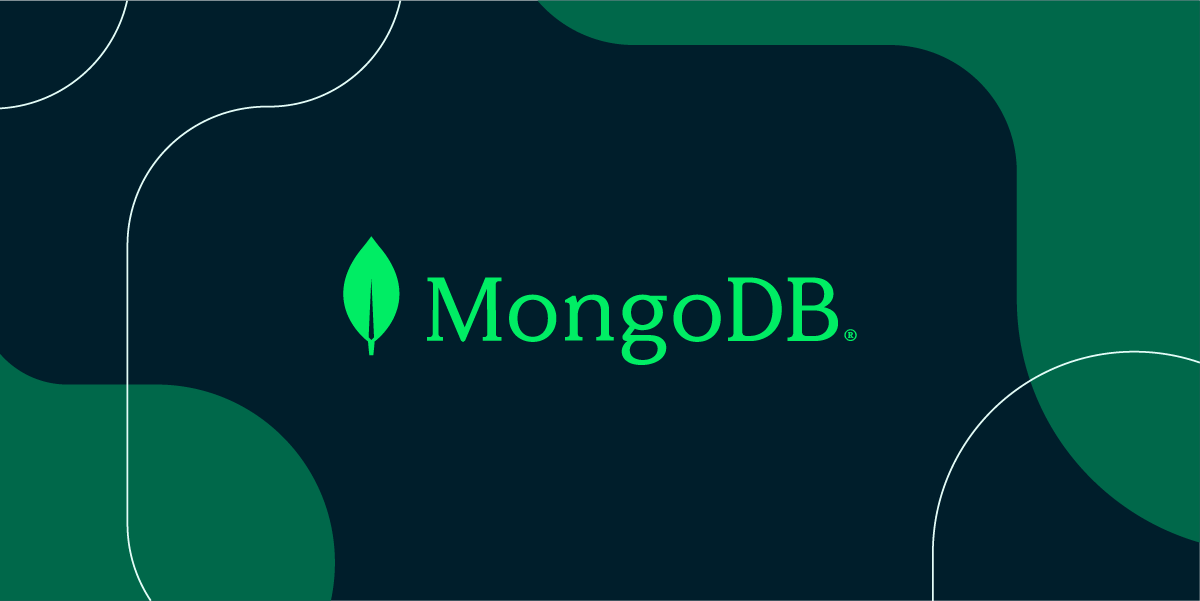
- Introduction
- Setting Up MongoDB and Mongoose
- Creating Data with MongoDB and Mongoose
- Reading Data with MongoDB and Mongoose
- Updating Data with MongoDB and Mongoose
- Deleting Data with MongoDB and Mongoose
- Class Material(s)
- Conclusion
- Resources
Introduction
MongoDB is a popular NoSQL database that uses documents instead of tables and rows to store data.
It is a highly scalable database that is great for storing large amounts of data, and it can be used for a wide range of applications.
It provides a way to interact with MongoDB by defining schemas and models for your data, making it easier to work with data in your application.
In this blog post, we'll explore CRUD operations using MongoDB and Mongoose. CRUD stands for Create, Read, Update, and Delete, and these are the four basic operations that are typically used to interact with a database.
Setting Up MongoDB and Mongoose
Before we can start using MongoDB and Mongoose, we need to set them up. Here are the steps to install and connect to MongoDB using Mongoose:
Installing MongoDB
To install MongoDB, you can follow the instructions on the MongoDB website. Once you have MongoDB installed, you can start the MongoDB server by running the mongod
command in your terminal.
Installing Mongoose
To install Mongoose, you can use the following command:
npm install mongoose
Connecting to MongoDB using Mongoose
To connect to MongoDB using Mongoose, we need to create a connection. Here's an example:
const mongoose = require("mongoose");
mongoose
.connect("mongodb://localhost/my_database", {
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false,
})
.then(() => {
console.log("Connected to MongoDB");
})
.catch((err) => {
console.error("Error connecting to MongoDB", err);
});
This code connects to a local MongoDB instance running on the default port 27017
. We also specify some options for the connection, such as useNewUrlParser
and useUnifiedTopology
, which are recommended options. Finally, we log a message to the console if the connection is successful, or an error message if there's a problem.
Creating Data with MongoDB and Mongoose
Now that we're connected to MongoDB using Mongoose, let's start creating data.
Creating a Mongoose Schema
A Mongoose schema defines the structure of the data we want to store in MongoDB. Here's an example:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
This code defines a bookSchema
with five fields: title
, author
, published
, pages
, and genre
. Each field has a specific data type, such as String
, Date
, or Number
.
Creating a Mongoose Model
A Mongoose model is a class that represents a collection of documents in MongoDB. Here's how to create a mongoose model:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
This code defines a Book
model using the bookSchema
we defined earlier.
Creating a MongoDB Document
To create a new document in MongoDB using Mongoose, we can create a new instance of our Book
model and save it. Here's how to create a document:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
const book = new Book({
title: "The Great Gatsby",
author: "F. Scott Fitzgerald",
published: new Date("1925-04-10"),
pages: 218,
genre: "Fiction",
});
book
.save()
.then(() => {
console.log("Book saved to database");
})
.catch((err) => {
console.error("Error saving book to database", err);
});
This code creates a new Book
instance with some sample data, and then saves it to the database using the save
method. We log a message to the console if the save operation is successful, or an error message if there's a problem.
Reading Data with MongoDB and Mongoose
Now that we know how to create data, let's look at how we can read data from MongoDB using Mongoose.
Finding All Documents in a Collection
To find all documents in a collection, we can use the find
method. Here's an example:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
Book.find()
.then((books) => {
console.log("All books:", books);
})
.catch((err) => {
console.error("Error finding books", err);
});
This code finds all documents in the books
collection and logs them to the console.
Finding a Specific Document by ID
To find a specific document by ID, we can use the findById
method. Here's an example:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
const id = "60745dd2e8c33c7d505d27f4";
Book.findById(id)
.then((book) => {
console.log("Book:", book);
})
.catch((err) => {
console.error("Error finding book", err);
});
This code finds a document in the books
collection with the specified ID and logs it to the console.
Finding Documents Using Query Conditions
To find documents using query conditions, we can use the find
method with a query object. Here's an example:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
const query = { author: "F. Scott Fitzgerald" };
Book.find(query)
.then((books) => {
console.log("Books by F. Scott Fitzgerald:", books);
})
.catch((err) => {
console.error("Error finding books", err);
});
This code finds all documents in the books
collection where the author
field is 'F. Scott Fitzgerald', and logs them to the console.
Updating Data with MongoDB and Mongoose
Now that we know how to create and read data, let's look at how we can update data in MongoDB using Mongoose.
Updating a Single Document
To update a single document, we can use the findOneAndUpdate
method. Here's an example:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
const id = "60745dd2e8c33c7d505d27f4";
const update = { title: "The Great Gatsby (Revised Edition)" };
Book.findOneAndUpdate({ _id: id }, update, { new: true })
.then((book) => {
console.log("Updated book:", book);
})
.catch((err) => {
console.error("Error updating book", err);
});
This code finds a document in the `books` collection with the specified ID and updates the `title` field. The `{ new: true }` option returns the updated document instead of the original document. We log the updated document to the console if the operation is successful, or an error message if there's a problem.
Updating Multiple Documents
To update multiple documents, we can use the `updateMany` method. Here's an example:
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
const query = { author: "F. Scott Fitzgerald" };
const update = { genre: "Classic Literature" };
Book.updateMany(query, update)
.then((result) => {
console.log(`Updated ${result.nModified} books`);
})
.catch((err) => {
console.error("Error updating books", err);
});
This code updates the genre
field of all documents in the books
collection where the author
field is 'F. Scott Fitzgerald'. We log the number of documents that were modified to the console if the operation is successful, or an error message if there's a problem.
Deleting Data with MongoDB and Mongoose
Now that we know how to create, read, and update data, let's look at how we can delete data from MongoDB using Mongoose.
Deleting a Single Document
To delete a single document, we can use the findOneAndDelete
method.
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
const id = "60745dd2e8c33c7d505d27f4";
Book.findOneAndDelete({ _id: id })
.then((book) => {
console.log("Deleted book:", book);
})
.catch((err) => {
console.error("Error deleting book", err);
});
This code finds a document in the books
collection with the specified ID and deletes it. We log the deleted document to the console if the operation is successful, or an error message if there's a problem.
Deleting Multiple Documents
To delete multiple documents, we can use the deleteMany
method.
const mongoose = require("mongoose");
const bookSchema = new mongoose.Schema({
title: String,
author: String,
published: Date,
pages: Number,
genre: String,
});
const Book = mongoose.model("Book", bookSchema);
const query = { author: "F. Scott Fitzgerald" };
Book.deleteMany(query)
.then((result) => {
console.log(`Deleted ${result.deletedCount} books`);
})
.catch((err) => {
console.error("Error deleting books", err);
});
This code deletes all documents in the books
collection where the author
field is 'F. Scott Fitzgerald'. We log the number of documents that were deleted to the console if the operation is successful, or an error message if there's a problem.
Class Material(s)
Conclusion
In this article, we've learned how to perform CRUD operations using MongoDB and Mongoose. We started by connecting to a MongoDB database using Mongoose and then went through examples of creating, reading, updating, and deleting data using Mongoose's built-in methods.
We also covered querying data using filters, sorting data, limiting and skipping results, and updating multiple documents at once.
With this knowledge, you should be able to work with MongoDB and Mongoose to build scalable and flexible applications.
If you're interested in learning more about MongoDB and Mongoose, be sure to check out the official documentation and experiment with different queries and methods to get a better understanding of how they work.