Introduction to Vue.js & Vue Options API
In this blog post, we’ll take a look at what Vue.js is, how to get started with it, and some of its core concepts.
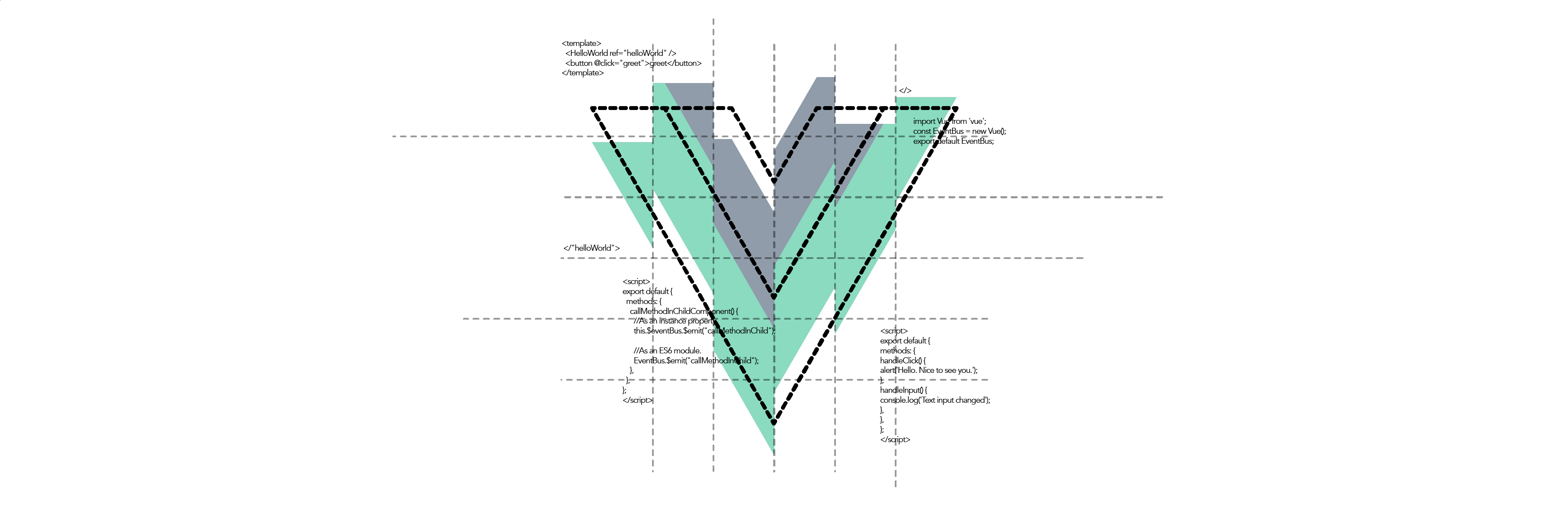
In this blog post, we will have a quick look at what Vue.js is and how to get started with it
- Introduction
- Getting Started
- Vue.js Core Concepts
- Working with Data
- Routing
- Introduction to Vue Options API
- Overview of the Options API
- How to Use the Options API
- Class Material(s)
- Conclusion
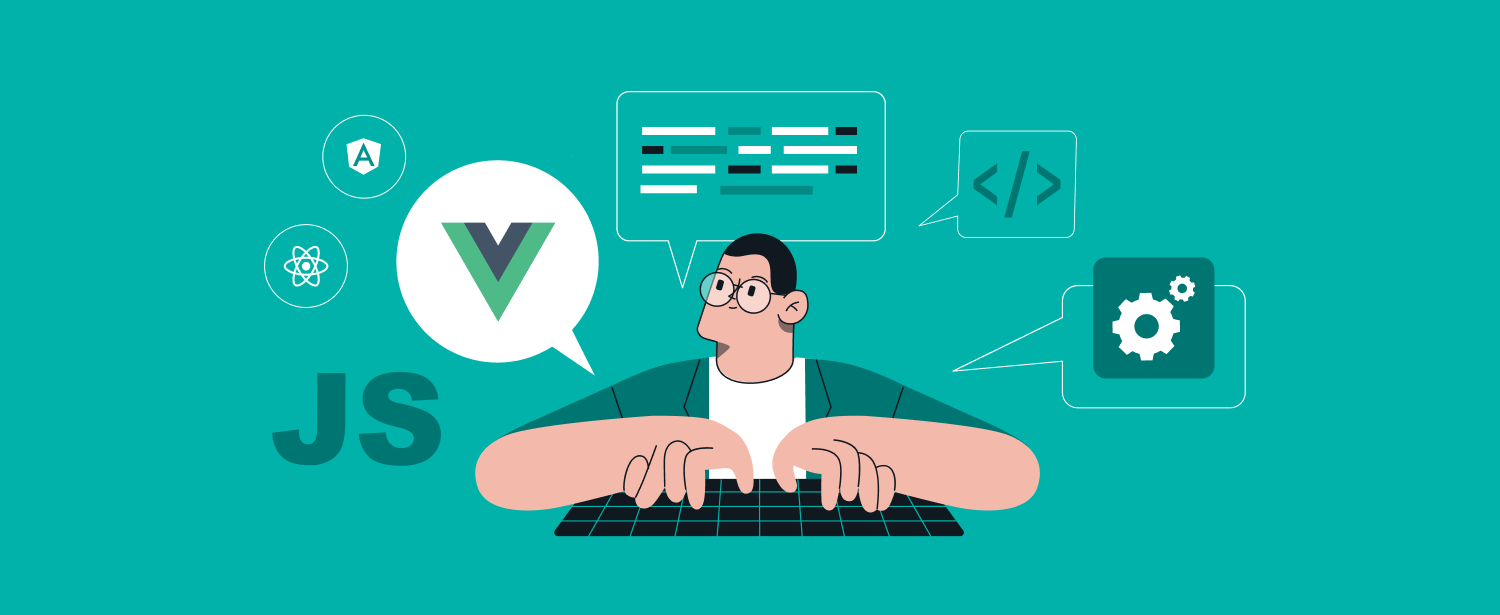
Introduction
Introduction to Vue.js?
Vue.js is a popular JavaScript framework for building user interfaces and single-page applications. It is designed to be fast, small, and easy to learn.
What is Vue.js?
Vue.js is an open-source JavaScript framework for creating user interfaces and single-page applications. Its main focus is on the view layer, which makes it easy to integrate with other projects and libraries.
💡
It is designed to be fast, lightweight, and easy to learn.
Benefits of Vue.js
Vue.js has several advantages over other frameworks and libraries.
- Lightweight
- Gentle Learning Curve
- Highly extensibility
- Reusability
- High Performance
- Good Documentation
- Community
- Simplicity
- Working with a Virtual DOM
Getting Started
Vue.js Installation
The first step to using Vue.js is to install it and the easiest way to do this is to use a package manager like npm or yarn. To install it using npm, run the following command:
npm install vue
Setting up a Vue.js Project
Once Vue.js is installed, the next step is to set up a project. To do this, create a new directory and create a file named “main.js”. Then, add the following code to the file:
// main.js
import Vue from 'vue'
new Vue({
el: '#app'
})
Next, create an HTML file and add the following code to it:
<!-- index.html -->
<div id="app"></div>
<script src="main.js"></script>
This will create a basic Vue.js project. From here, you can start building your application.
Vue.js Core Concepts
Components
Components are one of the core concepts of Vue.js. They are reusable pieces of code that can be used to create complex user interfaces. To create a component, use the Vue.component() method:
Vue.component('my-component', {
template: `<div>My Component</div>`
})
Reactivity
Vue.js uses a reactive data-binding system to keep the view and the data in sync. This means that when the data changes, the view will automatically update to reflect the changes.
Template Syntax
Vue.js uses an HTML-based template syntax to create user interfaces. This makes it easy to create dynamic content and bind data to HTML elements.
Directives
Directives are special attributes used to manipulate the DOM. For example, the v-if directive can be used to conditionally show or hide elements:
<div v-if="showElement">My Element</div>
Working with Data
Data Binding
Vue.js provides two-way data binding, which allows you to bind data to HTML elements and keep them in sync. This makes it easy to create dynamic user interfaces.
Events
Vue.js provides an event system that allows you to listen for and respond to user interactions. To listen for an event, use the v-on directive:
<button v-on:click="handleClick">Click Me</button>
State Management
Vue.js provides a state management system that makes it easy to manage data in complex applications. This can be done using the Vuex & Piana library.
Routing
Introduction to Routing
Routing is the process of mapping URLs to components. This allows you to create single-page applications that are more user-friendly.
Setting up Routes
To set up routes in Vue.js, use the vue-router library. This library provides an API for mapping URLs to components.
Introduction to Vue Options API
In the ever-evolving world of web development, it’s essential to stay up-to-date with the latest technologies. One of the most popular frameworks for developing web applications is Vue.js. It is a progressive JavaScript framework used to build user interfaces and single-page applications. With the arrival of Vue3, the framework has been updated with an options API.
What is Vue3 Options API?
The Vue3 Options API is a way of configuring a Vue instance. It is an object-based API that allows developers to configure components directly from the options object. This helps to provide a more straightforward and consistent way of configuring components.
Benefits of Using Vue3 Options API
The Vue3 Options API is a powerful API that can help developers to create complex applications. It helps to reduce the amount of code needed to develop a component. It also simplifies the process of configuring components. Additionally, the API provides more detailed documentation, making it easier for developers to understand the API and how to use it.
Overview of the Options API
The Vue3 Options API consists of several different properties and methods. The properties are used to set values for the component. The methods are used to call functions to perform specific actions on the component.
Properties
The properties available in the Vue3 Options API include:
- data: This is used to set the data for the component.
- props: This is used to set the props for the component.
- computed: This is used to set the computed properties for the component.
- methods: This is used to set the methods for the component.
Methods
The methods available in the Vue3 Options API include:
- $on: This is used to register an event listener.
- $off: This is used to unregister an event listener.
- $once: This is used to register an event listener that will be triggered only once.
- $emit: This is used to emit an event.
How to Use the Options API
The Vue3 Options API can be used in two ways: setting properties and calling methods.
Setting Properties
The properties can be set in the options object of the Vue instance. For example, the data property can be set like this:
data() {
return {
message: 'Hello World'
}
}
Calling Methods
The methods can be called on the Vue instance. For example, the $on method can be used to register an event listener like this:
const myEventListener = () => {
console.log('My event listener has been triggered!');
}
vm.$on('my-event', myEventListener)
Code Examples
This is a basic example of setting a property and calling a method:
Class Material(s)
Conclusion
In this blog post, we’ve taken a look at what Vue.js is and how to get started with it. We’ve also looked at some of its core concepts and how to work with data and routing. Here are some key takeaways:
- The Vue3 Options API is a powerful and versatile API that can be used to create complex applications
- The Options API helps to reduce the amount of code needed to develop a component and simplifies the process of configuring components.
- The Options API provides more detailed documentation, making it easier for developers to understand the API and how to use it.
Overall, the Vue3 Options API is a great way of configuring a Vue instance and can help developers to create robust applications quickly and efficiently.
Vue.js is lightweight, fast, and easy to learn. It also has a reactive data-binding system and a state management system that makes it easy to create complex applications.
Vue can also be used to build more complex web apps, and we have other meta-frameworks that makes it easier and faster to work with vue.js. e.g Nuxt.js
Resources for Learning Vue.js
If you’re looking to learn more about Vue.js, there are plenty of resources available.
The official Vue.js documentation is a great place to start. Additionally, there are many online courses and tutorials available.
We hope this blog post has given you a basic understanding of Vue.js and how to get started with it. Thanks for reading!