Using Array method on DOM, asynchronous javascript
By using array methods on the DOM, you can manipulate your web pages in a fast and efficient way
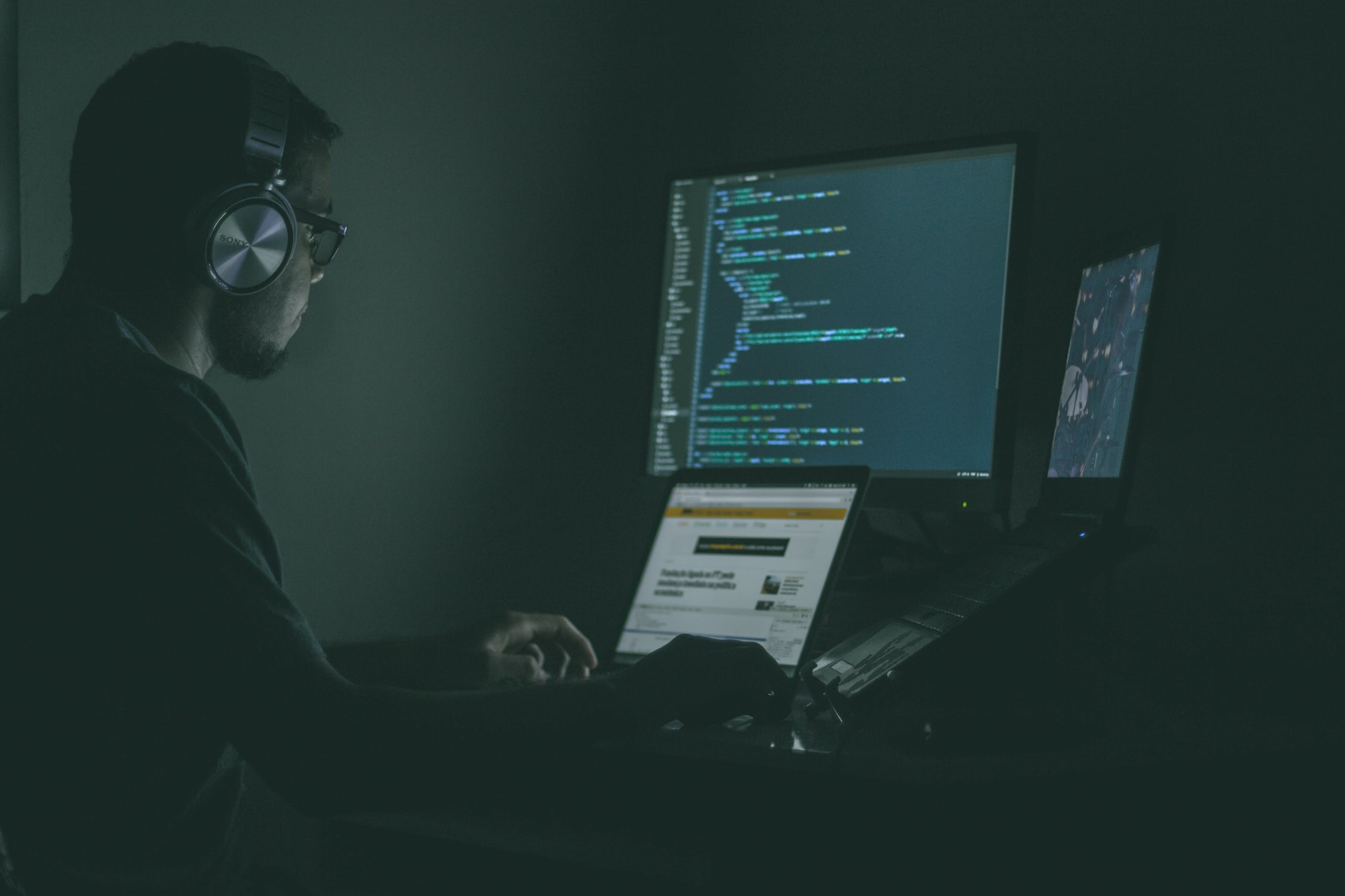
- Introduction
- Understanding the DOM
- Working with Arrays
- Array Methods for Manipulating the DOM
- Asynchronous JavaScript
- Using Asynchronous Array Methods on the DOM
- Class Material(s)
- Conclusion
Introduction
Asynchronous programming is becoming more and more prevalent in modern web development. One of the most popular tools for manipulating the Document Object Model (DOM) is JavaScript.
By using array methods on the DOM, you can manipulate your web pages in a fast and efficient way
In this post, we'll explore how to use array methods on the DOM and how to implement asynchronous programming in your JavaScript code.
Understanding the DOM
Before we dive into using array methods on the DOM, let's make sure we have a solid understanding of what the DOM is. The Document Object Model is a programming interface for web documents.
It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects. That way, programming languages can interact with the page.
Working with Arrays
Arrays are a fundamental part of programming in JavaScript. They allow you to store and manipulate collections of data. There are many methods available for manipulating arrays, including forEach()
, map()
, filter()
, and reduce()
. These methods allow you to iterate over arrays, modify their contents, and create new arrays from existing ones.
Array Methods for Manipulating the DOM
Now that we understand the basics of the DOM and arrays, let's see how we can use array methods to manipulate the DOM. In this section, we'll cover some commonly used array methods for working with the DOM.
Querying the DOM with Array.from()
To manipulate elements on a page, you first need to select them from the DOM. You can do this using the querySelectorAll()
method, which returns a NodeList. A NodeList is similar to an array, but it lacks many of the useful methods that arrays have.
To convert a NodeList into an array, you can use the Array.from()
method. Here's an example:
const divs = Array.from(document.querySelectorAll('div'));
Now you can use array methods on the divs
array to manipulate the selected elements.
Manipulating the DOM with forEach()
The forEach()
method allows you to iterate over an array and perform an operation on each element.
Here's an example of how to use it to change the text of each paragraph on a page:
const paragraphs = Array.from(document.querySelectorAll('p'));
paragraphs.forEach((paragraph) => {
paragraph.textContent = 'New Text';
});
Filtering the DOM with filter()
The filter()
method allows you to create a new array containing only the elements that match a certain condition. Here's an example of how to use it to select only the paragraphs that contain the word Lorem
:
const paragraphs = Array.from(document.querySelectorAll('p'));
const filteredParagraphs = paragraphs.filter((paragraph) => {
return paragraph.textContent.includes('Lorem');
});
Mapping the DOM with map()
The map()
method allows you to create a new array containing the results of applying a function to each element in the original array. Here's an example of how to use it to extract the text content of all the paragraphs on a page:
const paragraphs = Array.from(document.querySelectorAll('p'));
const paragraphText = paragraphs.map((paragraph) => {
return paragraph.textContent;
});
Asynchronous JavaScript
Asynchronous programming allows your code to perform tasks in the background without blocking the main thread.
This is especially useful for tasks that take a long time to complete, like fetching data from a server or performing complex calculations. Asynchronous programming in JavaScript is implemented using promises and async/await.
Promises
Promises
are a way to handle asynchronous operations in JavaScript. They represent a value that may not be available yet, but will be at some point in the future.
You can attach callbacks to a promise that will be executed once the value is available.
const url = 'https://jsonplaceholder.typicode.com/posts';
fetch(url)
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
})
.catch((error) => {
console.log(error);
});
Async/Await
Async/await
is a newer feature in JavaScript that allows you to write asynchronous code that looks more like synchronous code. It uses promises under the hood, but allows you to write code that is easier to read and understand.
async function getData() {
const url = 'https://jsonplaceholder.typicode.com/posts';
try {
const response = await fetch(url);
const data = await response.json();
console.log(data);
} catch (error) {
console.log(error);
}
}
Using Asynchronous Array Methods on the DOM
Now that we understand how to use array methods on the DOM and how to implement asynchronous programming in JavaScript, let's see how we can combine these concepts to create more efficient and powerful code.
Asynchronous forEach()
The `forEach()` method we covered earlier is a synchronous method, which means it will block the main thread while it performs its operations. This can be a problem if you're working with a large number of elements on a page. To solve this problem, you can use the forEach()
method from the async-array library.
const asyncForEach = require('async-array-foreach');
const paragraphs = Array.from(document.querySelectorAll('p'));
asyncForEach(paragraphs, async (paragraph) => {
const response = await fetch('https://example.com/api');
const data = await response.json();
paragraph.textContent = data.text;
});
Asynchronous map()
The map()
method we covered earlier is also a synchronous method. You can use the async-map library to perform asynchronous mapping operations on arrays. Here's an example:
const asyncMap = require('async-map');
const paragraphs = Array.from(document.querySelectorAll('p'));
asyncMap(paragraphs, async (paragraph) => {
const response = await fetch('https://example.com/api');
const data = await response.json();
return data.text;
}).then((paragraphText) => {
console.log(paragraphText);
});
Asynchronous filter()
The filter()
method we covered earlier is also a synchronous method. You can use the async-filter library to perform asynchronous filtering operations on arrays. Here's an example:
const asyncFilter = require('async-filter');
const paragraphs = Array.from(document.querySelectorAll('p'));
asyncFilter(paragraphs, async (paragraph) => {
const response = await fetch('https://example.com/api');
const data = await response.json();
return data.text.includes('Lorem');
}).then((filteredParagraphs) => {
console.log(filteredParagraphs);
});
Class Material(s)
Conclusion
Using array methods on the DOM can make your code more efficient and powerful.
When combined with asynchronous programming, you can create code that can handle large amounts of data and perform complex operations without blocking the main thread.
With the help of libraries like async-array
, async-map
, and async-filter
, you can easily implement asynchronous array methods on the DOM in your JavaScript code.